Parse CSV Files in Node.js with csv-parse
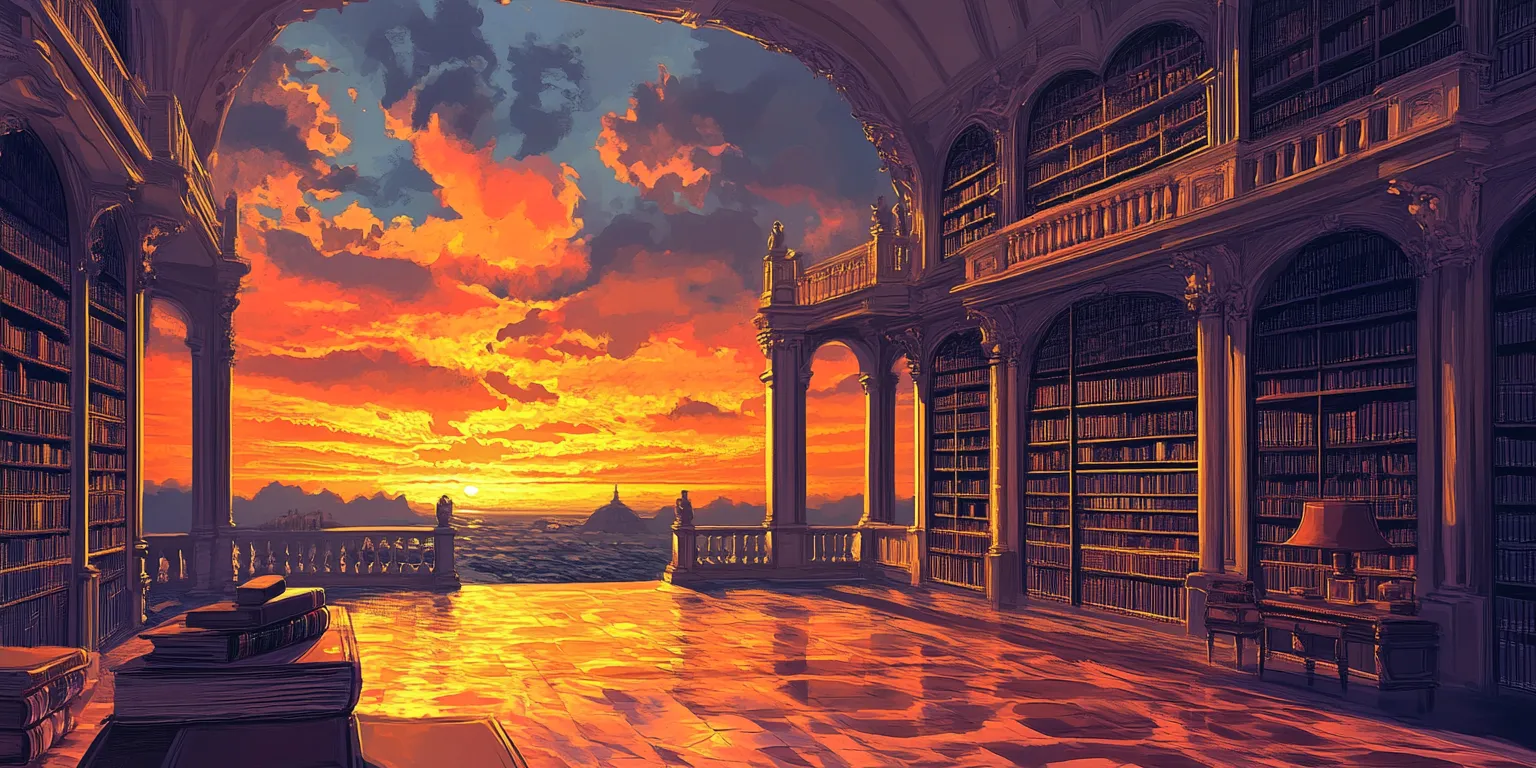
Imagine you’re building a tool that processes sales data from a spreadsheet exported as a comma-separated values (CSV) file. The file contains rows of customer names, purchase amounts, and dates—unstructured text that needs to be turned into usable data. Parsing this manually would be slow and error-prone, but Node.js offers a clean solution with the csv-parse library. This tool simplifies reading and processing CSV files, making it a practical choice for developers working with structured data. Here, you’ll learn how to use csv-parse to handle CSV files in Node.js, with examples to show it in action.
Setup and Installation
To start, you need to add csv-parse to your Node.js project. It’s part of the broader csv package, but you’ll focus on the parsing functionality.
- Install the package using npm:
npm install csv-parse
- Verify it’s added to your
package.json
dependencies.
This library works well with Node.js streams or direct string inputs, giving you flexibility depending on your use case—whether you’re reading a small file or streaming a large dataset.
Parsing a CSV String
Let’s begin with a simple case: parsing CSV data provided as a string. This is useful when the data is already in memory, like from an API response or a small test file.
Basic Parsing Example
Here’s how to parse a CSV string using csv-parse:
import { parse } from 'csv-parse/sync';
const csvData = `name,amount,date
Alice,50,2023-01-15
Bob,75,2023-02-10`;
const records = parse(csvData, {
columns: true,
skip_empty_lines: true
});
console.log(records);
This code outputs:
[
{ name: 'Alice', amount: '50', date: '2023-01-15' },
{ name: 'Bob', amount: '75', date: '2023-02-10' }
]
- The parse function comes from the synchronous module of csv-parse.
- The
columns: true
option tells it to use the first row as headers, creating an array of objects. - The
skip_empty_lines: true
option ignores any blank lines in the input.
This approach is straightforward and turns raw text into structured data you can work with immediately.
Reading a CSV File with Streams
For larger files, parsing the entire content into memory isn’t practical. Instead, you can use Node.js streams with csv-parse to process data line by line.
Streaming Example
Here’s an example reading from a file:
import { createReadStream } from 'fs';
import { parse } from 'csv-parse';
const fileStream = createReadStream('./sales-data.csv');
fileStream
.pipe(parse({ columns: true, skip_empty_lines: true }))
.on('data', (row) => {
console.log(row); // Process each row as it's parsed
})
.on('end', () => {
console.log('Finished parsing the file');
})
.on('error', (err) => {
console.error('Error:', err.message);
});
Assuming sales-data.csv
contains:
name,amount,date
Alice,50,2023-01-15
Bob,75,2023-02-10
The output logs each row individually:
{ name: 'Alice', amount: '50', date: '2023-01-15' }
{ name: 'Bob', amount: '75', date: '2023-02-10' }
Finished parsing the file
- The createReadStream function opens the file as a readable stream.
- The stream is piped into parse, which processes it incrementally.
- Event listeners (
data
,end
,error
) handle the rows, completion, and any issues.
This method keeps memory usage low, making it suited for large datasets like logs or exported reports.
Handling Custom CSV Formats
Not all CSV files follow a standard format. Some use different delimiters (like tabs or semicolons) or include comments. csv-parse lets you adjust for these variations.
Custom Delimiter Example
Suppose you have a file, custom-data.csv
, with semicolon separators:
name;amount;date
Alice;50;2023-01-15
Bob;75;2023-02-10
Here’s how to parse it:
import { createReadStream } from 'fs';
import { parse } from 'csv-parse';
createReadStream('./custom-data.csv')
.pipe(parse({ columns: true, delimiter: ';' }))
.on('data', (row) => {
console.log(row);
});
The delimiter: ';'
option overrides the default comma, correctly splitting the fields. You can also use comment: '#'
to skip lines starting with #
, or quote: '"'
to handle quoted values—options that make csv-parse adaptable to real-world files.
Note: Always check your CSV file’s structure beforehand to set the right options. Misconfigured settings can lead to parsing errors or unexpected results.
Processing Parsed Data
Once parsed, you’ll likely want to do something with the data—like filtering or calculating totals. Here’s a practical example building on the streaming approach.
Calculating Total Sales
Using the earlier streaming example:
import { createReadStream } from 'fs';
import { parse } from 'csv-parse';
let total = 0;
createReadStream('./sales-data.csv')
.pipe(parse({ columns: true, skip_empty_lines: true }))
.on('data', (row) => {
total += Number(row.amount); // Convert string to number
})
.on('end', () => {
console.log(`Total sales: $${total}`);
});
For the sample data, this outputs:
Total sales: $125
- The
Number()
conversion is needed since CSV values are strings by default. - This pattern lets you process data incrementally, keeping the code efficient.
This shows how csv-parse integrates into larger workflows, turning raw files into actionable insights.
Conclusion
Parsing CSV files in Node.js with csv-parse offers a reliable way to handle structured data, whether it’s a small string or a massive file. You can parse synchronously for quick tasks, use streams for efficiency, or tweak options for custom formats—all while keeping your code clean and focused. For developers dealing with data imports or exports, this library saves time and reduces complexity.
You might also like
Go-to-Market Strategy for SaaS: A Step-by-Step Guide
Learn how to build a go-to-market plan for your SaaS product. This guide covers finding your audience, setting prices, and launching step-by-step to grow your business.
Defining Your North Star Metric: A Step-by-Step Approach
Learn how to identify and implement your company's North Star Metric. This comprehensive guide will help you choose the right metric to drive growth and align your team.
The Power of Freemium: When (and When Not) to Offer a Free Plan
Explore when to implement a freemium model for your software product. Learn the benefits, challenges, and key factors to consider when deciding if a free plan is right for your business.
Canny Pricing: Is it worth it in 2025?
Canny is a tool that helps you manage your customer support. It's a great tool, but is it worth the price? Let's find out.