Match File Patterns Easily in Node.js with minimatch
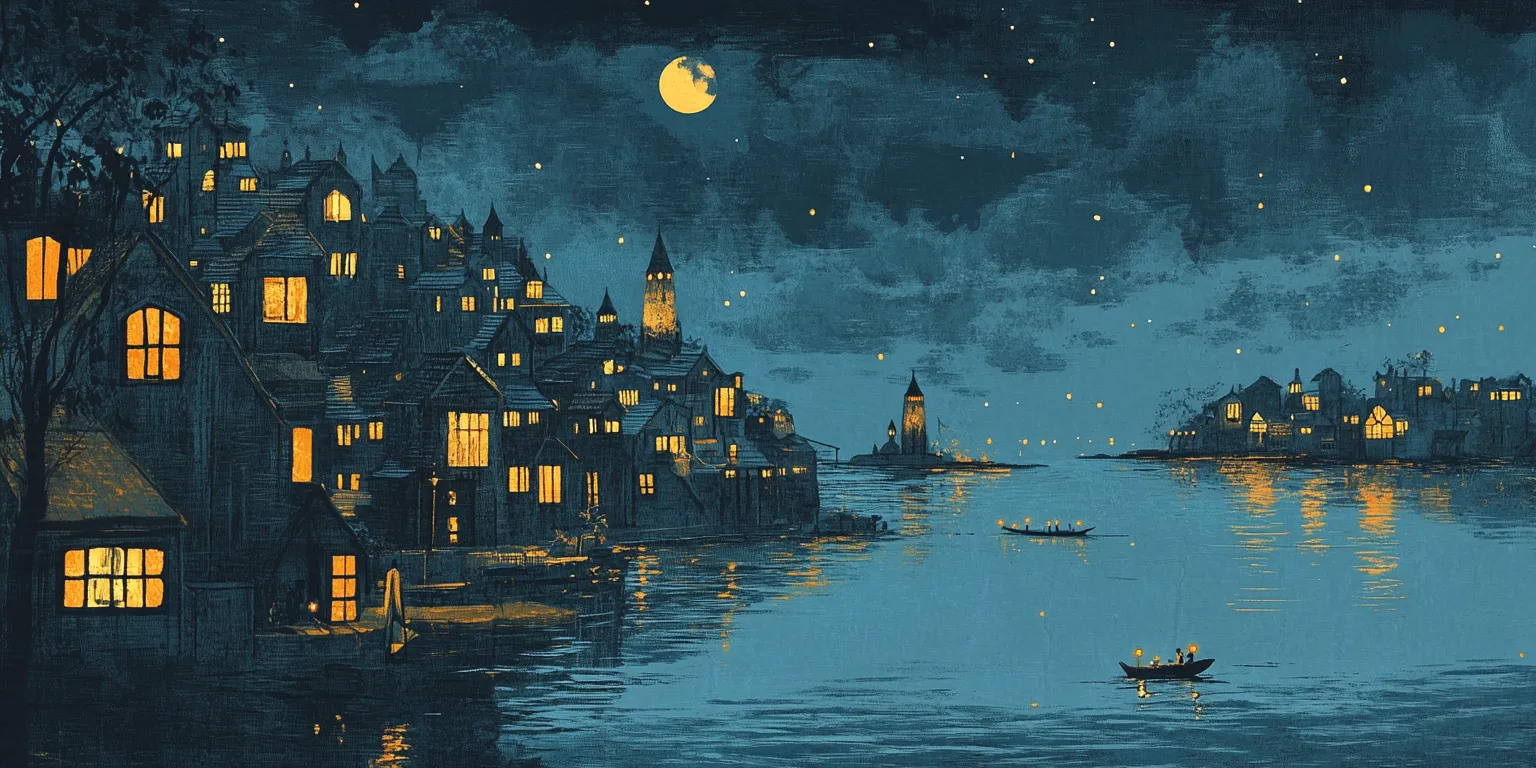
Imagine you’re building a tool to process files in a Node.js project, and you need to select only JavaScript files while ignoring those in a node_modules
folder. Manually filtering files can get messy fast. That’s where minimatch comes in—a simple, powerful library for matching file paths against patterns. It’s widely used in tools like Grunt and Gulp, making it a handy skill for developers working with file systems or build processes. This post walks you through how minimatch works, why it’s useful, and how to apply it effectively in your projects.
What is minimatch?
minimatch is a Node.js library that matches strings (like file paths) against glob patterns—think *.js
or src/**/*.ts
. It’s built to handle the kind of pattern matching you’d see in a terminal, but with extra control for programmatic use. If you’ve used globs before (e.g., ls *.txt
in Bash), minimatch brings that flexibility to JavaScript.
The library is lightweight and focused. You give it a string and a pattern, and it tells you if they match. This makes it perfect for tasks like filtering files, validating inputs, or setting up ignore rules.
Installing minimatch
To use it, first add it to your project with npm:
npm install minimatch
Then, bring it into your code:
const minimatch = require('minimatch');
That’s it—you’re ready to start matching patterns.
Core Concepts of minimatch
Before jumping into examples, let’s cover the basics of how minimatch operates. It uses glob syntax, which might feel familiar if you’ve worked with file paths or shell commands.
Glob Patterns Explained
A glob pattern is a string that defines a rule for matching. Here are the key pieces:
*
matches any sequence of characters in a single path segment (e.g.,*.js
matchesapp.js
but notsrc/app.js
).**
matches any sequence across multiple path segments (e.g.,src/**/*.js
matchessrc/app.js
andsrc/lib/util.js
).?
matches a single character (e.g.,file?.js
matchesfile1.js
but notfile12.js
).{a,b}
matches eithera
orb
(e.g.,file{1,2}.js
matchesfile1.js
orfile2.js
).!
at the start of a pattern negates it (e.g.,!node_modules/**
excludes files innode_modules
).
These building blocks let you craft precise rules for what to include or exclude.
How minimatch Processes Patterns
When you pass a string and a pattern to minimatch, it returns true
if they match and false
if they don’t. It’s case-sensitive by default but can be tweaked with options. The library also supports arrays of patterns, applying them in order—useful for combining includes and excludes.
Using minimatch in Practice
Let’s see minimatch in action with some real-world examples. These snippets show how to solve common file-matching tasks.
Matching Specific File Types
Suppose you want to filter a list of files to keep only JavaScript files:
const files = ['app.js', 'style.css', 'index.html', 'lib.js'];
const jsFiles = files.filter((file) => minimatch(file, '*.js'));
console.log(jsFiles); // ["app.js", "lib.js"]
Here, *.js
matches any file ending in .js
. The filter
method keeps only the matches.
Excluding Folders
Now, imagine you’re scanning a directory but want to skip node_modules
:
const paths = ['src/app.js', 'node_modules/lib.js', 'test/main.js'];
const pattern = '**/*.js';
const exclude = 'node_modules/**';
const filtered = paths.filter(
(path) => minimatch(path, pattern) && !minimatch(path, exclude)
);
console.log(filtered); // ["src/app.js", "test/main.js"]
First, **/*.js
grabs all .js
files anywhere in the structure. Then, !minimatch(path, exclude)
drops anything in node_modules
. Combining patterns like this keeps your logic clean.
Using Options for Flexibility
minimatch lets you tweak its behavior with an options object. For example, to make matching case-insensitive:
const file = 'APP.JS';
const matches = minimatch(file, '*.js', { nocase: true });
console.log(matches); // true
Without { nocase: true }
, this would return false
. Options like this give you control over edge cases.
Working with Arrays of Patterns
Sometimes one pattern isn’t enough. minimatch can handle arrays, applying them sequentially—later patterns can override earlier ones.
Example: Include and Exclude Together
Here’s how to match all .js
files except those in test
:
const files = ['app.js', 'test/main.js', 'lib.js'];
const patterns = ['*.js', '!test/**'];
const result = files.filter((file) => minimatch(file, patterns));
console.log(result); // ["app.js", "lib.js"]
The !test/**
pattern negates anything in test
, refining the initial *.js
match. This is great for complex rules.
Note: When using arrays, minimatch treats them as a single logical unit. The order matters—exclusions should come after inclusions.
Benefits of Using minimatch
By now, you can see how minimatch simplifies file pattern matching. It saves you from writing custom string-parsing logic, which can be error-prone and hard to maintain. Its glob syntax is intuitive if you’ve used shells or build tools, and the options let you adapt it to specific needs. Whether you’re filtering files, validating paths, or setting up a build script, it’s a reliable choice.
Conclusion
minimatch brings straightforward, powerful pattern matching to Node.js. With its glob syntax and flexible options, you can handle tasks like filtering files or excluding directories with minimal effort. It’s a small library that punches above its weight, especially for developers working on tools or file-heavy projects. Next time you need to match paths, give it a try—it’ll save you time and headaches.
You might also like
Parse CSV Files in Node.js with csv-parse
Learn how to use csv-parse in Node.js to read and process CSV files efficiently, with practical examples for developers.
Go-to-Market Strategy for SaaS: A Step-by-Step Guide
Learn how to build a go-to-market plan for your SaaS product. This guide covers finding your audience, setting prices, and launching step-by-step to grow your business.
Defining Your North Star Metric: A Step-by-Step Approach
Learn how to identify and implement your company's North Star Metric. This comprehensive guide will help you choose the right metric to drive growth and align your team.
The Power of Freemium: When (and When Not) to Offer a Free Plan
Explore when to implement a freemium model for your software product. Learn the benefits, challenges, and key factors to consider when deciding if a free plan is right for your business.