Simplify File System Tasks with fs-extra in Node.js
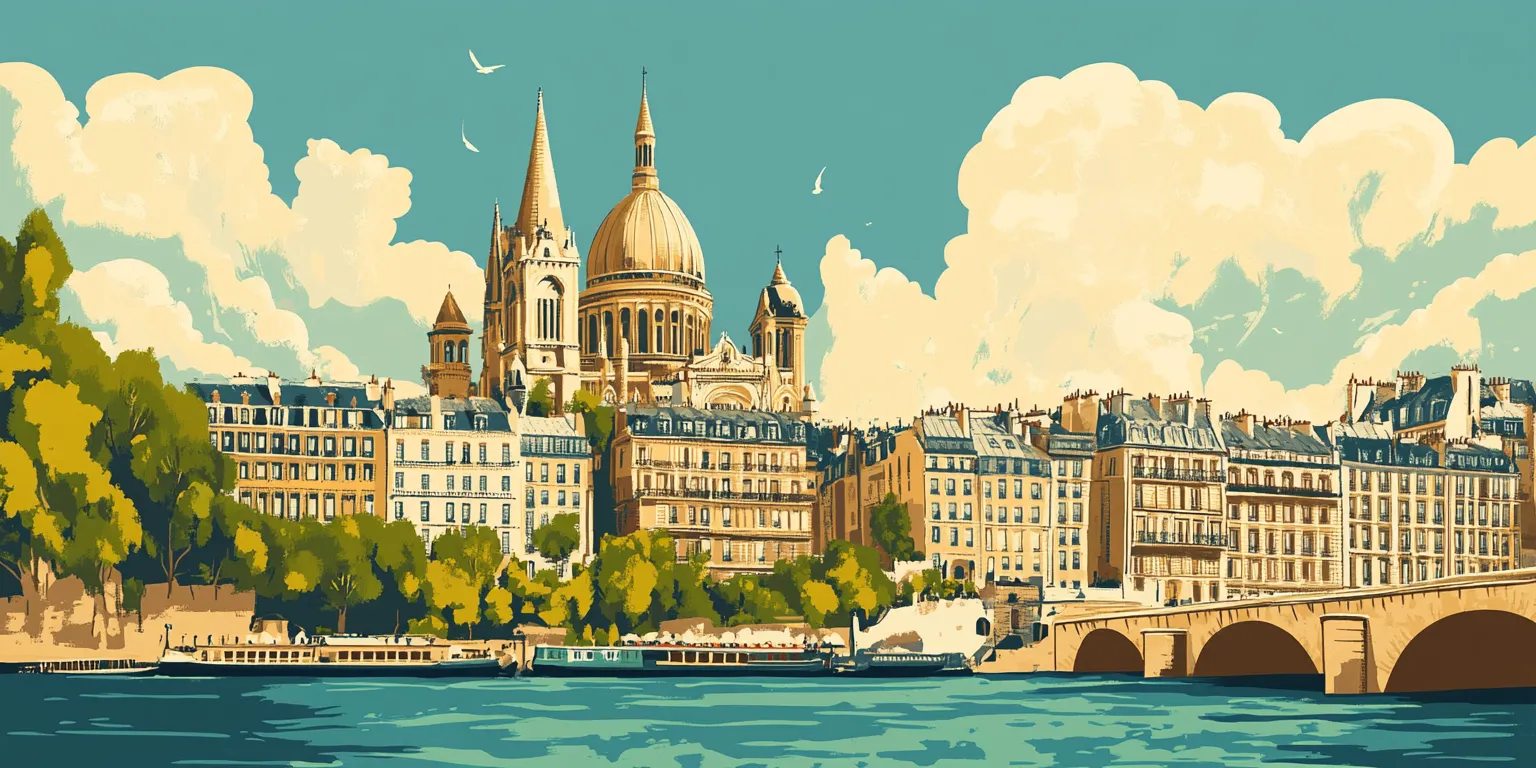
When building applications, especially those that interact with the file system, you’ll often find yourself needing more than what the standard Node.js fs
module offers. While fs
provides the basic tools for file and directory manipulation, it can become cumbersome when dealing with more complex operations. This is where fs-extra
shines. It’s a module that extends the functionality of the built-in fs
module, providing a more convenient and robust way to handle file system tasks.
Understanding the Basics
At its core, fs-extra
is a drop-in replacement for Node.js’s fs
module. It not only includes all the functions of the standard fs
module but also adds a variety of extra features that simplify common file system operations. These extra features include functions for creating directories recursively, copying files and directories, removing directories with all their contents, and much more. By using fs-extra
, you can write cleaner and more efficient code when working with the file system. It handles many of the edge cases and complexities that you might encounter when using the standard fs
module, making your development process smoother.
Getting Started with fs-extra
Before you can start using fs-extra
, you need to install it in your project. This is done using npm, the Node.js package manager. Open your terminal or command prompt, navigate to your project’s root directory, and run the following command:
npm install fs-extra
Once the installation is complete, you can import the module into your project and start using its functions. fs-extra
is designed to work seamlessly with both CommonJS and ESM import styles, so you can use whichever module system you prefer.
Common Uses of fs-extra
Let’s dive into some of the most useful functions that fs-extra
provides and how they can simplify your file system operations.
Creating Directories Recursively
One of the most common challenges when dealing with directories is creating nested structures. The standard fs
module’s mkdir
function can only create one directory at a time. If you need to create a nested directory structure, you have to manually create each directory in the hierarchy, which can be time-consuming and error-prone. fs-extra
provides the ensureDir
function, which simplifies this process by creating all necessary parent directories if they don’t already exist.
const fs = require('fs-extra');
async function createNestedDirectory() {
try {
await fs.ensureDir('./my/nested/directory/structure');
console.log('Nested directory structure created successfully.');
} catch (error) {
console.error('Error creating directory structure:', error);
}
}
createNestedDirectory();
In this example, the ensureDir
function will create the “my”, “nested”, “directory”, and “structure” folders, even if they don’t exist yet. This makes it easy to set up complex folder structures for your project.
Copying Files and Directories
Copying files and directories is another common task. With the standard fs
module, copying files involves reading the source file and writing its contents to the destination file. Copying directories is even more complex, requiring you to recursively copy each file and subdirectory. fs-extra
simplifies this with its copy
function, which can copy both files and directories with a single command.
const fs = require('fs-extra');
async function copyFilesAndDirectories() {
try {
await fs.copy('./source_file.txt', './destination_file.txt');
console.log('File copied successfully.');
await fs.copy('./source_directory', './destination_directory');
console.log('Directory copied successfully.');
} catch (error) {
console.error('Error copying files or directories:', error);
}
}
copyFilesAndDirectories();
This code will copy the content of source_file.txt
to destination_file.txt
. It will also copy the entire source_directory
and all its contents to destination_directory
. If the destination files or directories do not exist, they will be created. If they exist, they will be overwritten.
Removing Directories Recursively
Removing directories, especially those containing files and subdirectories, can be a tricky task with the standard fs
module. You would have to write code to recursively remove all the files and subdirectories before removing the parent directory. fs-extra
provides the remove
function, which can remove a directory and all of its contents with a single command.
const fs = require('fs-extra');
async function removeDirectoryRecursively() {
try {
await fs.remove('./my/nested/directory/structure');
console.log('Directory structure removed successfully.');
} catch (error) {
console.error('Error removing directory structure:', error);
}
}
removeDirectoryRecursively();
This code will remove the “my/nested/directory/structure” folder and all of its contents, making it easy to clean up temporary files and folders.
Checking if a Path Exists
Before performing any operations on a file or directory, it’s often necessary to check if it exists. fs-extra
provides the pathExists
function for this purpose, which returns a boolean indicating whether a given path exists.
const fs = require('fs-extra');
async function checkPathExistence() {
const path = './my/file.txt';
const exists = await fs.pathExists(path);
if (exists) {
console.log('The path exists.');
} else {
console.log('The path does not exist.');
}
}
checkPathExistence();
This code checks if the file my/file.txt
exists. It can be used for both files and directories, enabling you to write more robust code.
Writing and Reading JSON Files
fs-extra
simplifies working with JSON files. The writeJson
function takes a file path and a JavaScript object and writes the object to the file as a JSON string. Similarly, the readJson
function reads a JSON file and parses it into a JavaScript object.
const fs = require('fs-extra');
async function writeAndReadJsonFile() {
const data = { name: 'John', age: 30 };
try {
await fs.writeJson('./data.json', data);
console.log('JSON file written successfully.');
const readData = await fs.readJson('./data.json');
console.log('Data from JSON file:', readData);
} catch (error) {
console.error('Error writing or reading JSON file:', error);
}
}
writeAndReadJsonFile();
This code will create a file named data.json
and write the provided JavaScript object into it as JSON. It will then read the JSON file and output the parsed JavaScript object.
Going Further
A very powerful use case for fs-extra
is when you’re working with large documents and need to read, write, and manipulate them easily. This is actually a very common problem when building RAG applications that require working with all kinds of documents. If that’s something you’re interested in, you can check out my other blog post on creating AI agents in Node with AI SDK that walks you through how to do this and using Postgres for RAG use cases that walks you through how to store and query documents with Postgres.
Conclusion
As you can see, fs-extra
can be a very useful tool for simplifying file and directory operations in Node.js. It can make your code more readable, maintainable, and efficient. If you’re working with the file system in Node.js, you should definitely consider using fs-extra
.
You might also like
Automating Invoice Generation and Sending with Stripe
Learn how to automate the process of generating and sending invoices using Stripe and TypeScript.
Set Up a Message Queue with Postgres, PGMQ, and Docker
Learn how to set up a message queue similar to SQS with Postgres, PGMQ, and Docker to queue and process messages.
How to use Zod with Express for input validation?
Learn how to create an input validation middleware for Express using Zod.
Adding Coupons to Stripe Checkout
Learn how to add coupons to your Stripe checkout page when using the Stripe SDK.