Adding Coupons to Stripe Checkout
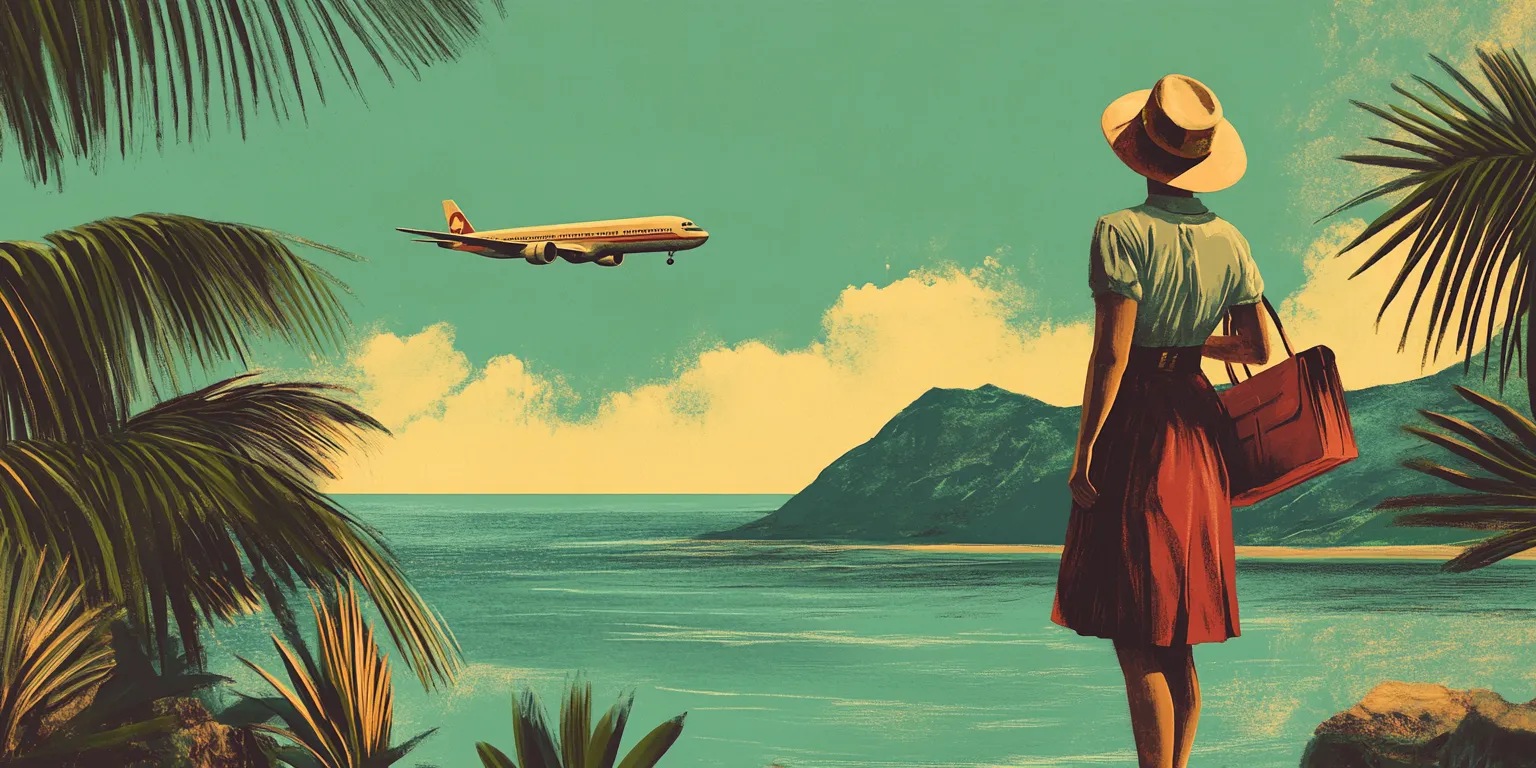
When you’re building a payment system for your SaaS product, giving users the option to use coupons can make your service more attractive. If you’re using Stripe for payments, you can easily set this up. Here’s how you can do it using TypeScript with the Stripe SDK.
Setting Up the Checkout Session
The first thing you need to do is create a Checkout Session. This is done using the stripe.checkout.sessions.create()
method from the Stripe SDK. When you’re creating this session, you need to make sure you include a setting that lets users apply coupons.
To allow coupons, you simply set the allow_promotion_codes
parameter to true
. This tells Stripe that it’s okay for customers to enter a promotion code during checkout. Here’s how you might write this in TypeScript:
import Stripe from 'stripe';
const stripe = new Stripe('your_stripe_secret_key', {
apiVersion: '2023-10-16'
});
const session = await stripe.checkout.sessions.create({
line_items: [
{
price: 'product_price_id',
quantity: 1
}
],
mode: 'payment',
allow_promotion_codes: true,
success_url: 'https://example.com/success',
cancel_url: 'https://example.com/cancel'
});
Pre-Applying a Coupon
If you want to make things even easier for your users, you can pre-apply a coupon for them. This means they don’t have to enter a code themselves. To do this, you add a discounts
array to your session creation call, and include the coupon ID in it.
For example, during Black Friday sales, you might want to have a direct link from a banner on your website that automatically applies a special discount. This can be a great way to boost sales during the holiday season. Here’s how you can pre-apply a coupon for such a scenario:
const session = await stripe.checkout.sessions.create({
line_items: [
{
price: 'product_price_id',
quantity: 1
}
],
mode: 'payment',
discounts: [
{
coupon: 'BLACK_FRIDAY_2025'
}
],
success_url: 'https://example.com/success',
cancel_url: 'https://example.com/cancel'
});
In this example, when a user clicks on the Black Friday banner, they are taken directly to the checkout page with the BLACK_FRIDAY_2025
coupon already applied, making the shopping experience smoother and more enticing.
Things to Keep in Mind
It’s important to know that Stripe only lets you use one coupon or promotion code per Checkout Session. So, if you’re planning on offering multiple discounts, you’ll need to think about how you want to handle that.
In addition, you can also use the stripe.promotionCodes.list()
method to list all the promotion codes that are available to you. This can be useful if you want to display a list of all the available coupons to the user.
If you also want to learn more about Stripe, you can check out our other blog posts on Accepting Payments with Stripe and Remix and Creating Automated Invoices with Stripe.
You might also like
Accepting Payments with Stripe and Remix
Learn how to accept payments with Stripe and Remix. We'll walk you through the process of setting up Stripe and Remix to accept payments.
How to Enrich Customer Data with LLMs and Web Crawling
Learn how to use LLMs and Puppeteer to crawl customer websites and enrich their data within your SaaS product.
Creating AI Agents in Node Using the AI SDK
Learn how to create AI agents in Node using the AI SDK to automate workflows and tasks.
What Does "Cleaned" Mean in Mailchimp?
An explanation of what "cleaned" means in Mailchimp and how to manage your email list.