Add Color to Your Terminal Output with Chalk in Node.js
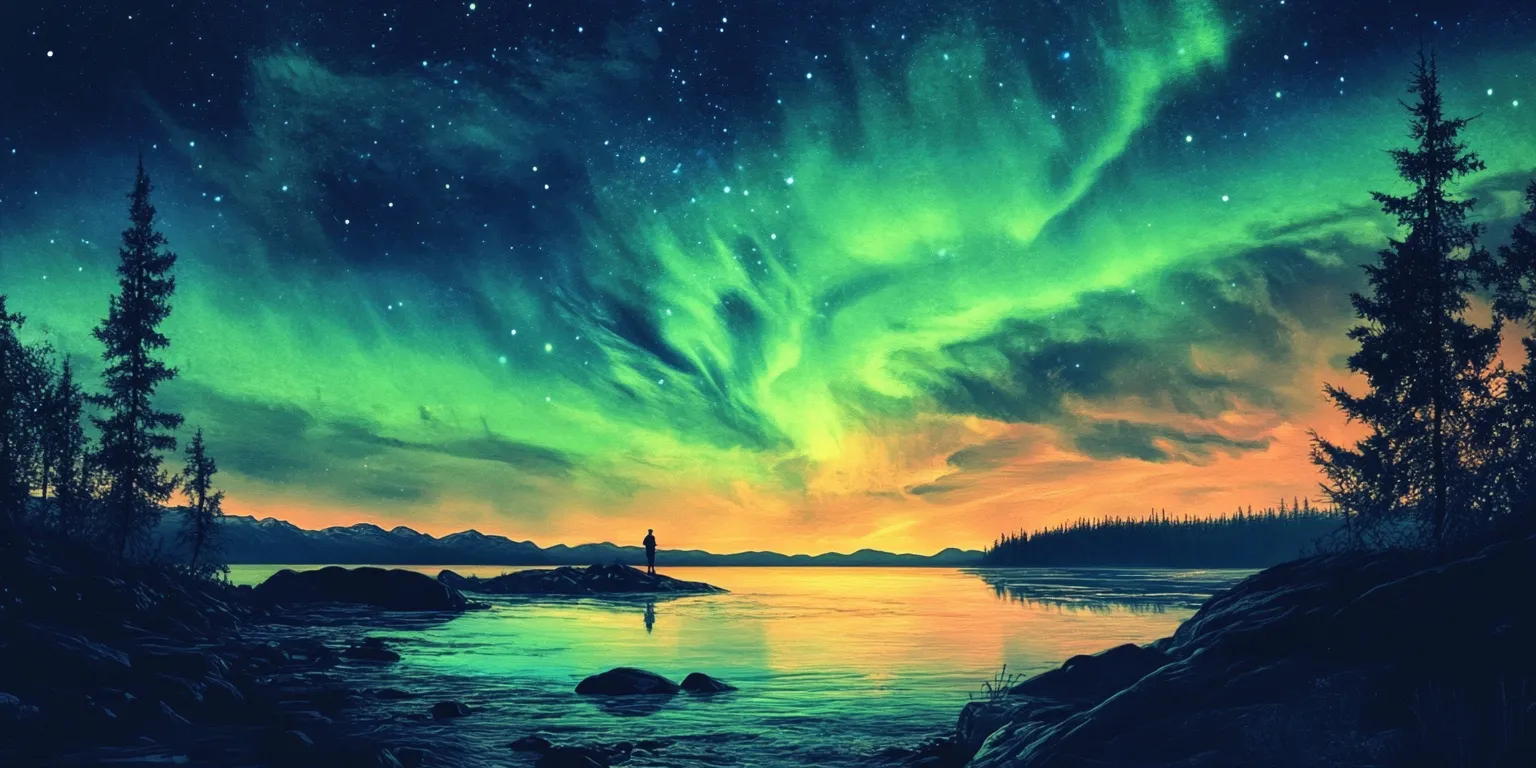
When you run a Node.js script in your terminal, the output is often plain text in a single color. Imagine building a command-line tool where logs, errors, and success messages all look the same—hard to scan quickly. Adding color can make these outputs easier to read and understand. This is where Chalk, a popular Node.js library, comes in. It lets you style terminal text with colors and formatting, improving the experience for developers and users alike. Let’s explore how to use it effectively.
Getting Started with Chalk
Chalk is a simple tool for adding color and styles to terminal output. It’s lightweight, easy to set up, and works well with Node.js projects.
Installation
To use Chalk, you need to install it in your project. Open your terminal, navigate to your project folder, and run:
npm install chalk
This adds Chalk to your node_modules
and updates your package.json
.
Basic Usage
Once installed, you can import Chalk into your script and start applying styles. Here’s a quick example:
const chalk = require('chalk');
console.log(chalk.blue('This text is blue!'));
console.log(chalk.red('This text is red!'));
Run this with node yourfile.js
, and you’ll see the text in blue and red. Chalk provides functions like blue
and red
that wrap your strings in the right ANSI escape codes for terminal colors.
Applying Colors and Styles
Chalk offers more than just basic colors. You can combine styles, use background colors, and apply formatting like bold or underline.
Common Color Options
Chalk supports standard terminal colors. Here are some examples:
chalk.green('Success')
– Green text, often used for success messages.chalk.yellow('Warning')
– Yellow text, good for warnings.chalk.red('Error')
– Red text, typically for errors.
Try this in a script:
const chalk = require('chalk');
console.log(chalk.green('Task completed'));
console.log(chalk.yellow('Check your input'));
console.log(chalk.red('Something went wrong'));
Each line stands out, making it clear what’s happening at a glance.
Adding Background Colors and Styles
You can also set background colors or add effects like bold or italic. Use properties like bg
for backgrounds and chain styles together:
const chalk = require('chalk');
console.log(chalk.bgBlue.white('White on blue'));
console.log(chalk.bold.red('Bold red text'));
console.log(chalk.underline.green('Underlined green'));
In this code:
bgBlue.white
sets a blue background with white text.bold.red
makes the text bold and red.underline.green
adds an underline to green text.
Note: Not all terminals support every style (like italic or underline). Test your output to confirm it works in your environment.
Chaining Styles
Chalk lets you chain multiple styles in a single call. This keeps your code clean:
const chalk = require('chalk');
console.log(chalk.bold.underline.blue('Bold, underlined, and blue'));
The order doesn’t matter—Chalk applies all styles correctly.
Practical Use Cases
Colorful output isn’t just for looks—it solves real problems in development and tooling.
Improving Logs
When debugging, colored logs help you spot issues faster. Here’s an example of a simple logging function:
const chalk = require('chalk');
function log(type, message) {
if (type === 'success') {
console.log(chalk.green(message));
} else if (type === 'warn') {
console.log(chalk.yellow(message));
} else if (type === 'error') {
console.log(chalk.red(message));
}
}
log('success', 'Server started on port 3000');
log('warn', 'Database connection slow');
log('error', 'Failed to load config');
This makes it easy to skim the output and focus on what needs attention.
Building CLI Tools
If you’re creating a command-line interface (CLI), Chalk adds polish. Imagine a script that checks system status:
const chalk = require('chalk');
function checkStatus() {
const cpuOk = true;
const memoryOk = false;
console.log('System Status:');
console.log(`CPU: ${cpuOk ? chalk.green('OK') : chalk.red('FAIL')}`);
console.log(`Memory: ${memoryOk ? chalk.green('OK') : chalk.red('FAIL')}`);
}
checkStatus();
The output uses green for “OK” and red for “FAIL,” giving users a clear visual cue.
Handling Edge Cases
Chalk is simple, but there are a few things to keep in mind.
Terminal Support
Some terminals (like older Windows Command Prompt versions) don’t handle colors well. Chalk detects this and disables colors by default if needed. To force colors, set the FORCE_COLOR
environment variable:
FORCE_COLOR=1 node yourfile.js
Performance
For small scripts, Chalk’s overhead is tiny. In a loop with thousands of logs, though, formatting can slow things down slightly. Use it where it adds value, like user-facing output, not deep debug logs.
Note: If colors don’t show up, check your terminal settings or try a modern one like iTerm2 or Windows Terminal.
Conclusion
Chalk makes terminal output more readable and engaging with minimal effort. By adding colors and styles, you can highlight key information, improve debugging, and create better CLI tools. It’s a small addition that brings clear benefits to your Node.js projects.
Going Further
- Check the Chalk documentation for advanced features like custom themes or RGB colors.
- Read about ANSI escape codes to understand how terminal styling works under the hood.
You might also like
Match File Patterns Easily in Node.js with minimatch
Learn how to use minimatch in Node.js to match file patterns with glob syntax, simplifying file filtering and path validation.
Parse CSV Files in Node.js with csv-parse
Learn how to use csv-parse in Node.js to read and process CSV files efficiently, with practical examples for developers.
Go-to-Market Strategy for SaaS: A Step-by-Step Guide
Learn how to build a go-to-market plan for your SaaS product. This guide covers finding your audience, setting prices, and launching step-by-step to grow your business.
Defining Your North Star Metric: A Step-by-Step Approach
Learn how to identify and implement your company's North Star Metric. This comprehensive guide will help you choose the right metric to drive growth and align your team.